TP1 : Local Illumination Models
- Program sources
- GLSL Specifications
- OpenGL 3.2 API Quick Reference Card
The deadline for giving back TP1 is: Monday, November 25, 2013 (23:59, European Time)
Warmup
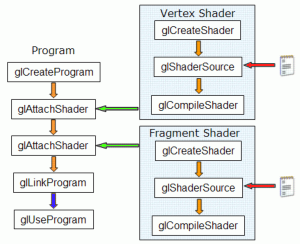
(from LightHouse3D, A. R. Fernandes)
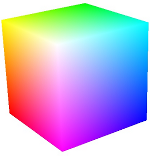
Test the smoothColor shader. You should get the picture of a cube. The colors are interpolated between the vertices. You can edit the shader and reload it at any time using 'CTRL+L'. The code for all the shaders is inside the files .vert for the the vertex shaders, and inside .frag for the fragment shaders (all of them in the folder qviewer/shaders). There is a menu to let you select between the different shaders.
Shader management is encapsulted in the GQShaderManager class. As illustrated in the diagram on the right, this class loads and compiles the vertex and fragment shaders, then link them together. In the source code for the method drawScene in the class Scene, you will see an example of using GQShaderManager. Once the program is loaded and activated, we can initialize the uniform variables, especially the transformation matrices.
Next, observe the code for creating and drawing a Vertex Array Object (VAO) in the Cube class. The Vertex Array Object will send for display, in a single instruction, the entire geometry (vertices, colors and normals) to the GPU. A first method, createVAO, creates this VAO from the geometry. The second method, draw, activates the VAO and sends it to the GPU. In the remainder of the practicals, we will use the class GQVertexBuffer to encapsulate these calls.
Phong model
The model is illuminated by a light source, of color lalight_color. The local illumination from this light source is made of ambiant lighting ca, diffuse lighting cd and specular lighting cs. Assuming N is the normal at the point P where we want to compute illumination, L is the direction towards the light source from point P and V is the direction towards the view point from point P, then:
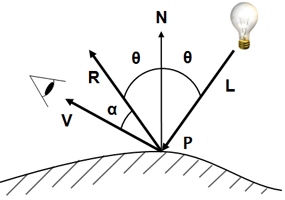
ca = ka * ma * light_color
with ka the ambient reflection coefficient and ma the ambient color for the material.
cd = kd * md * N.L * light_color
with kd the diffuse reflection coefficient and md the diffuse color of the material.
cs = ks * ms * light_color * (max(R.V,0))^s
with ks the specular reflection coefficient, ms the specular color of the material and s the shininess of the object.
The object color is then ca + cd + cs.
Gouraud shading (per vertex)
Start with the phongVertex shader, and modify it so that it computes the local illumination on the Lemming, using Phong model, computed for each vertex.
In order to understand well the different components of lighting, write the shader so that it can display each component separately: only ambient lighting, then diffuse lighting, then specular lighting:
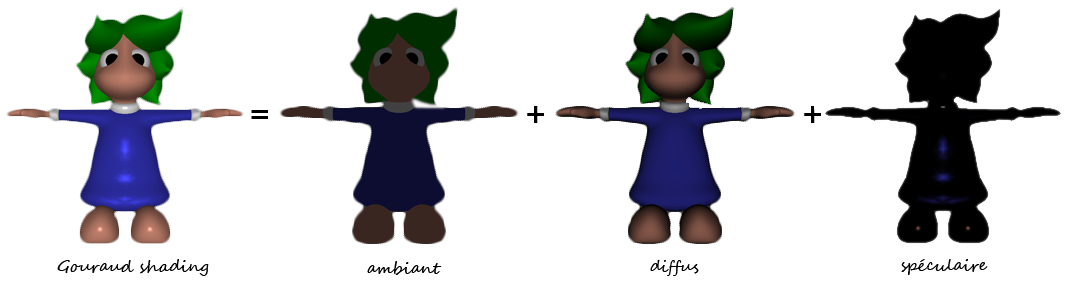
Phong shading (per fragment)
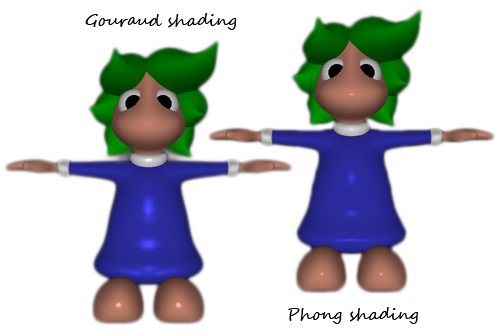
Now change the shader so that they compute shading for each fragment instead of each vertex. You will need to transfer the vectors L, N and V from the vertex shader to the fragment shader. Remember the normalization!
How does the shading change with fragment shading?
What about the extra cost for shading?
Blinn-Phong BRDF
Change the shaders so that you now have a Blinn-Phong material. This time, the specular component should be:
cs = ks * ms * ls * (max(N.H,0))^s
where H is the half-vector between the view vector V and the light source L.
You should get almost the same result as the classical Phong if you multiply the shininess exponent by 4.
Toon shading
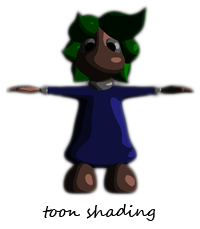
Toon shading is a shading model that generates pictures that look like toons. The key idea is to threshold the shading values computed, in order to get ranges of uniform colors.
.The color is computed based on the angle between the normal N and the light vector L, through the dot product N.L, and with multiband thresholding.
Create a new pair of shaders toon that implement this first step of non-photorealistic rendering (we'll do more about this during practical 3.